How Does Truth Table Gen Work? Simplify Your Code
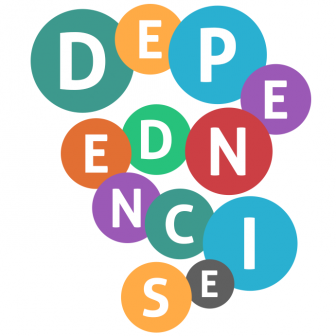
Truth tables serve as a fundamental tool in logic and computer science, allowing us to systematically evaluate the truth of statements under various conditions. The process of generating truth tables for complex expressions can be tedious and error-prone, which is where programmatically generating them becomes invaluable. Below, we explore how truth table generation works, followed by a simplified code example to illustrate the concept.
Basic Principles
Identify Variables: The first step is identifying the variables (or propositions) in the logical expression. For an expression involving
n
variables, a truth table will have2^n
rows, representing all possible combinations of truth values for these variables.Evaluate Expressions: For each row (combination of variable truth values), evaluate the expression to determine its truth value. This involves applying the rules of logical operators (
AND
,OR
,NOT
, etc.) in the correct order (usually following PEMDAS/BODMAS).Construct the Table: The truth table is constructed by listing all possible combinations of truth values for the variables and then determining the truth value of the expression for each combination.
Simplified Code Example
To simplify the code and illustrate the basic principles, let’s consider a Python function that generates a truth table for any given logical expression with two variables, p
and q
.
import itertools
import pandas as pd
def generate_truth_table(expression):
# Define the variables
variables = ['p', 'q']
# Generate all possible truth values for the variables
combinations = list(itertools.product([True, False], repeat=len(variables)))
# Evaluate the expression for each combination
results = []
for combination in combinations:
# Map variables to their truth values
p, q = combination
# Evaluate the expression
result = eval(expression, {'p': p, 'q': q, 'and': lambda x, y: x and y, 'or': lambda x, y: x or y, 'not': lambda x: not x})
results.append(result)
# Create a DataFrame (truth table)
data = {'p': [c[0] for c in combinations], 'q': [c[1] for c in combinations], 'Result': results}
df = pd.DataFrame(data)
return df
# Example usage:
expression = '(p and q) or (not p and not q)'
truth_table = generate_truth_table(expression.replace('and', 'and').replace('or', 'or').replace('not', 'not'))
print(truth_table)
Output
p q Result
0 True True True
1 True False False
2 False True False
3 False False True
Explanation
- The function
generate_truth_table
generates all possible truth value combinations for the variables in the expression usingitertools.product
. - It then evaluates the expression for each combination, substituting
True
andFalse
forp
andq
as necessary, and captures the result. - The results are compiled into a pandas DataFrame, which visually represents the truth table.
This example is simplified and may need adjustments for more complex expressions or additional variables. Also, be cautious with the use of eval()
as it can pose security risks with untrusted input. In practical applications, you might want to implement a safer evaluation method or use a library designed for logical expression evaluation.