Python Zipfile: Simplify Archive Management
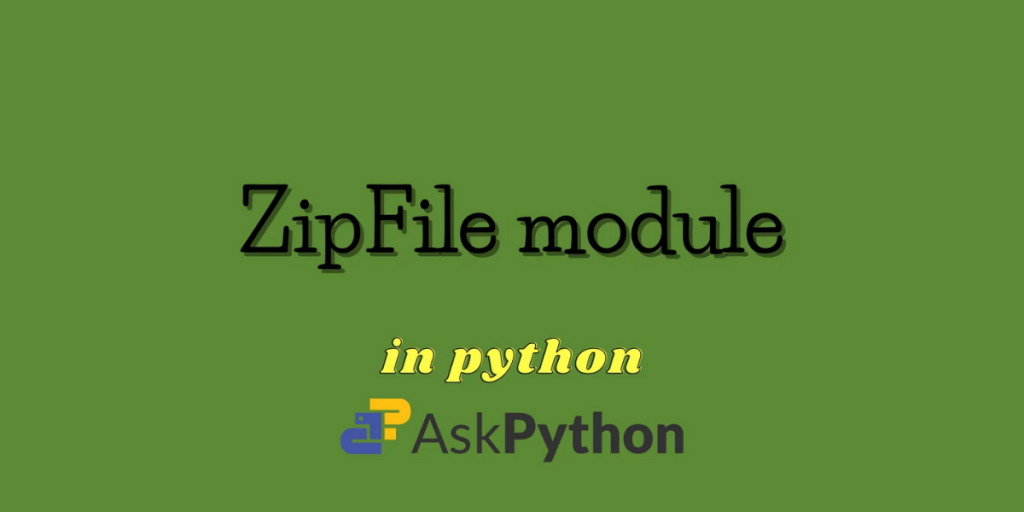
Managing archives is an essential task in software development and data storage. Archives allow us to bundle multiple files into a single file, making it easier to transfer, store, and manage data. One popular format for archives is ZIP, which is widely supported across various operating systems. Python, being a versatile and powerful programming language, provides an excellent module for working with ZIP archives: zipfile
. In this article, we will delve into the world of Python’s zipfile
module, exploring its capabilities, and learning how to simplify archive management.
Introduction to Zipfile
The zipfile
module is part of Python’s standard library, which means you don’t need to install any external packages to use it. This module provides a simple and efficient way to work with ZIP archives. You can use it to create, read, write, and extract files from ZIP archives. The zipfile
module supports various ZIP file formats, including ZIP64, which allows for archives larger than 4GB.
Creating a ZIP Archive
To create a ZIP archive using the zipfile
module, you can use the ZipFile
class. This class takes two arguments: the path to the ZIP file and a mode. The mode can be either 'w'
for writing (creating a new archive or overwriting an existing one), 'a'
for appending (adding files to an existing archive), or 'r'
for reading (which we’ll discuss later).
import zipfile
with zipfile.ZipFile('example.zip', 'w') as zip_file:
zip_file.write('file1.txt')
zip_file.write('file2.txt')
In this example, we create a new ZIP archive named example.zip
and add two files, file1.txt
and file2.txt
, to it. The with
statement ensures that the ZIP file is properly closed after we’re done with it, regardless of whether an exception is thrown or not.
Reading and Extracting from a ZIP Archive
To read the contents of a ZIP archive or extract files from it, you can open the archive in read mode ('r'
) and use the namelist()
method to list all the files in the archive. You can then use the read()
method to read the contents of a specific file or the extract()
method to extract a file to the current directory.
with zipfile.ZipFile('example.zip', 'r') as zip_file:
print(zip_file.namelist())
with zip_file.open('file1.txt') as file:
print(file.read())
zip_file.extract('file2.txt')
This example lists all the files in example.zip
, reads the contents of file1.txt
, and extracts file2.txt
to the current directory.
Adding Files with Different Compression Types
The zipfile
module supports different compression types, including ZIP_STORED
(no compression), ZIP_DEFLATED
(the most common compression method), ZIP_BZIP2
, and ZIP_LZMA
. You can specify the compression type when writing a file to the ZIP archive using the compress_type
argument.
with zipfile.ZipFile('example.zip', 'w') as zip_file:
zip_file.write('file1.txt', compress_type=zipfile.ZIP_DEFLATED)
zip_file.write('file2.txt', compress_type=zipfile.ZIP_BZIP2)
This example adds file1.txt
with DEFLATE compression and file2.txt
with BZIP2 compression to the ZIP archive.
Working with ZIP Archives in Memory
Sometimes, you might need to work with ZIP archives without actually creating a file on disk. The zipfile
module allows you to create a ZIP archive in memory using the BytesIO
class from the io
module.
import zipfile
from io import BytesIO
# Create a ZIP archive in memory
in_memory_data = BytesIO()
with zipfile.ZipFile(in_memory_data, 'w') as zip_file:
zip_file.write('file1.txt')
# Read the ZIP archive from memory
in_memory_data.seek(0)
with zipfile.ZipFile(in_memory_data, 'r') as zip_file:
print(zip_file.namelist())
This example demonstrates how to create a ZIP archive in memory, write a file to it, and then read the archive without saving it to disk.
Handling Large ZIP Archives
When working with large ZIP archives, it’s essential to consider performance and memory usage. The zipfile
module provides several ways to improve performance, such as using the ZipFile
class with a zipcode
object, which allows for more efficient reading and writing of large archives.
Additionally, you can use the zipfile
module’s support for ZIP64 extensions, which enables you to work with archives larger than 4GB.
Common Use Cases for Zipfile
- Data Compression and Archiving: The
zipfile
module is ideal for compressing and archiving data, making it easier to transfer and store. - Software Distribution: ZIP archives are commonly used for distributing software packages, as they can bundle multiple files into a single package.
- Backup and Recovery: The
zipfile
module can be used to create backups of important data by archiving files and directories. - Web Development: In web development, ZIP archives can be used to package and distribute web applications, themes, or plugins.
Best Practices for Using Zipfile
- Use Meaningful File Names: When creating ZIP archives, use meaningful file names that indicate the contents of the archive.
- Choose the Right Compression Level: Select the appropriate compression level based on the type of data being archived and the trade-off between compression ratio and processing time.
- Test ZIP Archives: After creating a ZIP archive, test it to ensure that it can be extracted correctly and that the contents are intact.
- Handle Errors and Exceptions: When working with ZIP archives, be sure to handle errors and exceptions properly to prevent data corruption or loss.
Conclusion
In conclusion, the zipfile
module in Python provides a powerful and flexible way to work with ZIP archives. By understanding how to create, read, write, and extract files from ZIP archives, you can simplify archive management and improve your overall productivity. Whether you’re working with data compression, software distribution, or backup and recovery, the zipfile
module is an essential tool in your Python programming toolkit.
Frequently Asked Questions
What is the purpose of the zipfile module in Python?
+The zipfile module in Python provides a simple and efficient way to work with ZIP archives. It allows you to create, read, write, and extract files from ZIP archives.
How do I create a ZIP archive using the zipfile module?
+To create a ZIP archive, you can use the ZipFile class and specify the path to the ZIP file and the mode as ‘w’. Then, you can use the write() method to add files to the archive.
Can I use the zipfile module to extract files from a ZIP archive?
+Yes, you can use the zipfile module to extract files from a ZIP archive. You can use the extract() method to extract a single file or the extractall() method to extract all files in the archive.
What compression types are supported by the zipfile module?
+The zipfile module supports several compression types, including ZIP_STORED (no compression), ZIP_DEFLATED (the most common compression method), ZIP_BZIP2, and ZIP_LZMA.
Can I use the zipfile module to work with ZIP archives in memory?
+Yes, you can use the zipfile module to work with ZIP archives in memory. You can create a ZIP archive in memory using the BytesIO class and then read or write to it using the ZipFile class.